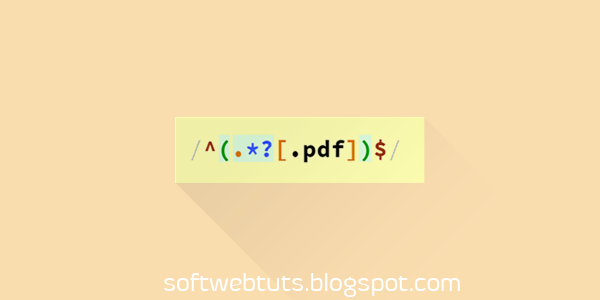
Regular expressions basically are patterns that are used to match combinations of characters in strings and today I am going to tell you or provide you a complete guide about regular expressions.
That's why I called this article "Regular Expression Tutorial Complete Guide".
In other words a regular expression is an object that describes a pattern of characters. Regular expression also pronounced as a regex or regexp is a sequence of characters that defines a search pattern usually search patterns that are used to search or find anything in a string.
Regular Expressions are special text strings for describing the search pattern. You may call regular expression a wild card. You may have seen or you may have used, if you are a computer user *.txt to search all the files with txt extension in file manager. basically this wild card *.txt is a regular expression.
Regular expressions are used in every programming language but in this article we will talk about JavaScript or jQuery that most of the web designers and web developers mostly use.
Why should I learn Regular Expressions?
Today most of the popular text editors for example notepad++ also uses regular expression functionality to replace or remove any particular text or string from the given text.It these regex or regular expression can be very much beneficial for you if you have a ling script of text and you want to remove or replace specified text or string.
In JavaScript regular expressions also works as objects. These regex or patterns can be used with the following functions are methods in JavaScript and jQuery.
Javascript Functions or Methods to Use Regex:
- test(), exec() methods of RegExp
- match(), matchAll(), replace(), search() and split() methods of string
Methods/Functions that use regular expressions:
Method | Description |
---|---|
exec() | Executes a search for a match in a string. It returns an array of information or null on a mismatch. |
test() | Tests for a match in a string. It returns true or false. |
match() | Returns an array containing all of the matches, including capturing groups, or null if no match is found. |
matchAll() | Returns an iterator containing all of the matches, including capturing groups. |
search() | Tests for a match in a string. It returns the index of the match, or -1 if the search fails. |
replace() | Executes a search for a match in a string, and replaces the matched substring with a replacement substring. |
split() | Uses a regular expression or a fixed string to break a string into an array of substrings. |
In this article we will only have discussion about JavaScript regular expressions.
Method to Create a regular expression in JavaScript:
Literally there are two ways that can be used to make regular expressions in JavaScript or jQuery programming language and that two ways are described as under:var regex = /[a-z-Z-A]/;
var regex = new RegExp('[a-z-Z-A]');
Flags in Regular Expressions:
Regular expressions consists of some flags which have functionalities like global search, case insensitive search, multi-line search etc.List of flags in regular expressions:
This is a list of flags that can be used in regular expressions to perform specified functionalities with their descriptions you can read the description and understand the functionality of a specified flag and use it in your regular expressions.These flags can be used at the end of your regular expressions after the ending slash. Eg: /(.*?)/g
Flag | Description |
---|---|
g | g or Global regex flag is used to search string globally and without this flag in your regular expression you will only get first match. |
m | m or Multi Line slide is used to search are matches friend multiple times globally. In multi line flag ^ and $ are used to match start and end of line respectively. |
i | i or intensitive search of this flag is case insensitive. This flag do not have difference between X ( capital X ) or x ( small x ) |
s | This flag enables "dot-all" mode that allows . in regular expression to match new line escape sequence ( \n ). |
u | This flag enables full Unicode support in your regular expressions and enables correct processing of surrogate pairs. |
y | This is a sticky mode regular expression flag that is used to search at the exact position in string. |
x | x or extended flag ignore all the white spaces that are matched in your string. |
X | X or eXtra slag disallows meaningless escapes sequences that you have used in your regular expression. |
u | u or unicode regular expression flag allow your regular expression to be matched with full Unicode. |
U | u on Ungreedy regular expression flag makes quantifiers used in your regex lazy. |
J | J or Jchanged flag allow duplicate sub-pattern names in regex. |
D | this flag uses Dollar sign ( $ ) to match the end of pattern. |
The regular expression flags that are listed in the above table are mostly used by every programming language but some of the flags are not supported now-a-days.
Now let me give you the example of using methods and functions that uses regular expressions in JavaScript or jQuery.
Use of Regex in Javascript Methods:
Regex Search in a string: str.match:
This method finds all the matches in regex in a string.Example Code:
var str = "You can, you can do it.";
alert(str.match(/you/gi));
Output:
You,you // You,you (an array of 2 substrings that match)
Regex Replacement in String: str.replace:
This method is used to replace something in a string.Example Code:
var str = "You can, you can do it.";
alert(str.replace(/You/ig, "I"));
Output:
I can, I can do it.
Testing regex: regexp.test:
This method looks for at least one match in a string, if found and returns "true" otherwise it will return "false".Example code:
var str = "You can, you can do it.";
var regexp = /you/i;
alert(regexp.test(str));
Output:
true
Regular Expression or Regex Cheat Sheet:
Most of people use shortcuts to learn anything so for your ease I have created a quick cheat sheet of regex.These are regular expression tokens with their brief descriptions.
Anchors | Description |
---|---|
^ | Start of string, or start of line in multi-line pattern |
\A | Start of string |
$ | End of string, or end of line in multi-line pattern |
\Z | End of string |
\b | Word boundary |
\B | Not word boundary |
\< | Start of word |
\> | End of word |
Quantifiers | Description | ||
---|---|---|---|
* | 0 or more | {3} | Exactly 3 |
+ | 1 or more | {3,} | 3 or more |
? | 0 or 1 | {3,5} | 3, 4 or 5 |
Groups & Ranges | Description |
---|---|
. | Any character except new line (\n) |
(a|b) | a or b |
(...) | Group |
(?:...) | Passive (non-capturing) group |
[abc] | Range (a or b or c) |
[^abc] | Not (a or b or c) |
[a-q] | Lower case letter from a to q |
[A-Q] | Upper case letter from A to Q |
[0-7] | Digit from 0 to 7 |
\x | Group/subpattern number "x" |
Escape/Meta Sequences | Description |
---|---|
\c | Control character |
\s | White space |
\S | Not white space |
\d | Digit |
\D | Not digit |
\w | Word |
\W | Not word |
\x | Hexadecimal digit |
\O | Octal digit |
Character Classes | Description |
---|---|
\ | Escape following character |
\Q | Begin literal sequence |
\E | End literal sequence |
Common Metacharacters | Description | ||
---|---|---|---|
^ | [ | . | $ |
{ | * | ( | \ |
+ | ) | | | ? |
< | > |
Flags or Pattern Modifiers | Description |
---|---|
g | Global match |
i * | Case-insensitive |
m * | Multiple lines |
s * | Treat string as single line |
x * | Allow comments and whitespace in pattern |
e * | Evaluate replacement |
U * | Ungreedy pattern |
POSIX | Description |
---|---|
[:upper:] | Upper case letters |
[:lower:] | Lower case letters |
[:alpha:] | All letters |
[:alnum:] | Digits and letters |
[:digit:] | Digits |
[:xdigit:] | Hexadecimal digits |
[:punct:] | Punctuation |
[:blank:] | Space and tab |
[:space:] | Blank characters |
[:cntrl:] | Control characters |
[:graph:] | Printed characters |
[:print:] | Printed characters and spaces |
[:word:] | Digits, letters and underscore |
Special Characters | Description |
---|---|
\n | New line |
\r | Carriage return |
\t | Tab |
\v | Vertical tab |
\f | Form feed |
\xxx | Octal character xxx |
\xhh | Hex character hh |
String | Replacement |
---|---|
$n | nth non-passive group |
$2 | "xyz" in /^(abc(xyz))$/ |
$1 | "xyz" in /^(?:abc)(xyz)$/ |
$` | Before matched string |
$' | After matched string |
$+ | Last matched string |
$& | Entire matched string |
Assertions | Description |
---|---|
?= | Lookahead assertion |
?! | Negative lookahead |
?<= | Lookbehind assertion |
?!=or ?<! | Negative lookbehind |
?> | Once-only Subexpression |
?() | Condition [if then] |
?()| | Condition [if then else] |
?# | Comment |
Regex Cheat Sheet PDF:
If you want to download the regular expression cheat sheet in PDF version it is also available you can download it from the link provided below.Download
Javascript Regex Examples:
So after I have provided you regular expression or regex cheat sheet and now I am going to provide you some examples of regular expressions that you can use in practicing regular expressions.var regex;
/* matching a specific string */
regex = /hello/; // looks for the string between the forward slashes (case-sensitive)... matches "hello", "hello123", "123hello123", "123hello"; doesn't match for "hell0", "Hello"
regex = /hello/i; // looks for the string between the forward slashes (case-insensitive)... matches "hello", "HelLo", "123HelLO"
regex = /hello/g; // looks for multiple occurrences of string between the forward slashes...
/* wildcards */
regex = /h.llo/; // the "." matches any one character other than a new line character... matches "hello", "hallo" but not "h\nllo"
regex = /h.*llo/; // the "*" matches any character(s) zero or more times... matches "hello", "heeeeeello", "hllo", "hwarwareallo"
/* shorthand character classes */
regex = /\d/; // matches any digit
regex = /\D/; // matches any non-digit
regex = /\w/; // matches any word character (a-z, A-Z, 0-9, _)
regex = /\W/; // matches any non-word character
regex = /\s/; // matches any white space character (\r (carriage return),\n (new line), \t (tab), \f (form feed))
regex = /\S/; // matches any non-white space character
/* specific characters */
regex = /[aeiou]/; // matches any character in square brackets
regex = /[ck]atherine/; // matches catherine or katherine
regex = /[^aeiou]/; // matches anything except the characters in square brackets
/* character ranges */
regex = /[a-z]/; // matches all lowercase letters
regex = /[A-Z]/; // matches all uppercase letters
regex = /[e-l]/; // matches lowercase letters e to l (inclusive)
regex = /[F-P]/; // matches all uppercase letters F to P (inclusive)
regex = /[0-9]/; // matches all digits
regex = /[5-9]/; // matches any digit from 5 to 9 (inclusive)
regex = /[a-zA-Z]/; // matches all lowercase and uppercase letters
regex = /[^a-zA-Z]/; // matches non-letters
/* matching repetitions using quantifiers */
regex = /(hello){4}/; // matches "hellohellohellohello"
regex = /hello{3}/; // matches "hellooo" and "helloooo" but not "helloo"
regex = /\d{3}/; // matches 3 digits ("312", "122", "111", "12312321" but not "12")
regex = /\d{3,7}/; // matches digits that occur between 3 and 7 times (inclusive)
regex = /\d{3,}/; // matches digits that occur at least 3 times
/* matching repetitions using star and plus */
regex = /ab*c/; // matches zero or more repetitions of "b" (matches "abc", "abbbbc", "ac")
regex = /ab+c/; // matches one or more repetitions of "b" (matches "abc", "abbbbc", but not "ac")
/* matching beginning and end items */
regex = /^[A-Z]\w*/; // matches "H", "Hello", but not "hey"
regex = /\w*s$/; // matches "cats", "dogs", "avocados", but not "javascript"
/* matching word boundaries
positions of word boundaries:
1. before the first character in string (if first character is a word character)
2. after the last character in the string, if the last character is a word character
3. between two characters in string, where one is a word character and the other isn't */
regex = /\bmeow\b/; // matches "hey meow lol", "hey:meow:lol", but not "heymeow lol"
/* groups */
regex = /it is (ice )?cold outside/; // matches "it is ice cold outside" and "it is cold outside"
regex = /it is (?:ice )?cold outside/; // same as above except it is a non-capturing group
regex = /do (cats) like taco \1/; // matches "do cats like taco cats"
regex = /do (cats) like (taco)\? do \2 \1 like you\?/; // matches "do cats like taco? do taco cats like you?"
//branch reset group (available in Perl, PHP, R, Delphi... commented out because this is a js file)
// regex = /(?|(cat)|(dog))\1/; // matches "catcat" and "dogdog"
/* alternative matching */
regex = /i like (tacos|boba|guacamole)\./; // matches "i like tacos.", "i like boba.", and "i like guacamole."
/* forward reference (available in Perl, PHP, Java, Ruby, etc... commented out because this is a js file) */
// regex = /(\2train|(choo))+/; // matches "choo", "choochoo", "chootrain", choochootrain", but not "train"
/* lookaheads */
regex = /z(?=a)/; // positive lookahead... matches the "z" before the "a" in pizza" but not the first "z"
regex = /z(?!a)/; // negative lookahead... matches the first "z" but not the "z" before the "a"
/* lookbehinds */
regex = /(?<=[aeiou])\w/; // positive lookbehind... matches any word character that is preceded by a vowel
regex = /(?<![aeiou])\w/; // negative lookbehind... matches any word character that is not preceded by a vowel
/* functions I find useful */
regex.test("hello"); // returns true if found a match, false otherwise
regex.exec("hello"); // returns result array, null otherwise
"football".replace(/foot/,"basket"); // replaces matches with second argument
Regular Expression or Regex Test:
You can test your regular expressions by using test method or function in JavaScript and also there are plenty of online and offline software that allows you to test your regular expressions in real-time.If you are new and you are trying to learn and test your regular expressions you can use the following tools that can help you and testing your regular expressions.